Player Movement
For the movement of the platformer game, I wanted the movement to feel responsive and consistent.
The current movement features are:
- A/D for left/right movement
- Space for jump and double jump
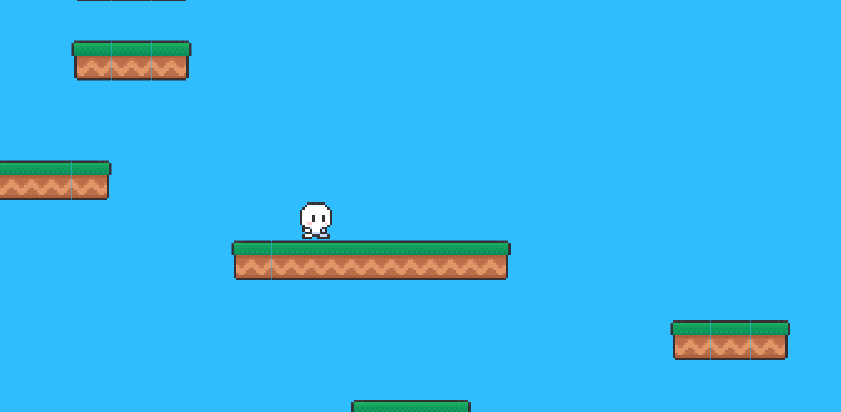
Since this is a platformer game, I wanted to movement speed to be consistent and at a comfortable pace for players to be able to control.
The hardcoded keys that players control are A/D for left/right movement and is based on vector 3 transform.position.
From the movement speed is also multiplied by Time.deltaTime to ensure the speed of the player remains the same despite the frame rate.
transform.position += new Vector3(movement, 0, 0) * Time.deltaTime * MovementSpeed;
For the jump and double jump, I wanted the jump to feel more 'natural' meaning that rather than just directly transforming the position of the player up, the mass of the player should be accounted for so the jump physics is based on addForce and ForceMode2D.impulse that adds instantaneous force.
rb.AddForce(new Vector2(0, JumpHeight), ForceMode2D.Impulse);
For the double jump one of the issues was that it wasn't really a double jump - you could just keep pressing space and infinitely jumping and whenever the I pressed space, it couldn't distinguish whether to use first jump or the double jump which lead to an interesting bug - The super jump
Just the simple fix for this was to instead use two keys for jump (W and Space) which would cause double inputs and result in higher jumps, add two boolean checkers, isGrounded for the first jump and isAir for the second jump to distinguish the what jump to apply and make the only jump button to be space.
if (Input.GetButtonDown("Jump") && isGrounded == true) { *Jump* inAir = true; } if (Input.GetButtonDown("Jump") && isGrounded == false && inAir == true) { *Jump* inAir = false; }
One of the things I didn't like about the initial jump, was it felt floaty and the falling time is the same time as jump height time. To improve the jump, I implemented a fall multiplier and low jump multiplier which would manipulate the gravity physics. This improved jumping physics is based on this video
Basically how the fall multiplier method is used is if the player velocity.y is below a certain value (e.g 0 and less) and physic gravity would multiply by a custom fallMultiplier value and that value would increase over time. Similarly the low jump multiplier which makes the jump height correlated to how long they press the jump key, uses the same idea
if (rb.velocity.y < -1) // fall/lowJump multiplier { rb.velocity += Vector2.up * Physics2D.gravity.y *(fallMultiplier -1) * Time.deltaTime; */or (lowJump -1) }
Player Feedback:
Something that Ian suggested to be was a Coyote time to give players extra leniency when jumping off the very edge and when playing the game I also did notice that I would fall off instead of jumping very frequently.
To fix this I added a hangTime variable which would give players the ability to jump even after they had fallen off the edge. This value is currently set to 0.2s
if (isGrounded) { hangCounter = hangTime; isHanging = false; } else { hangCounter -= Time.deltaTime; trail.time = 1; } if (Input.GetButtonDown("Jump") && hangCounter > 0f && isHanging == false) { *Jump* }
However the implementation of the coyote time did add back the super jump (which I'm trying to fix again currently, it not in the current build right now)
Another feedback I received was about the double jump timing
The changes I made to the jump was decreases the fall multiplier and as well as increase the double jump height to make the double jump more noticeable. I have also implemented a ghost falling effect when the player is falling
Get wow i can't believe it's another jump platformer
wow i can't believe it's another jump platformer
just another jump platformer :)
Status | In development |
Author | eddiecUTAS |
Genre | Action, Platformer |
Tags | 2D, Difficult, Pixel Art, Unity |
Languages | English |
More posts
- Documentation + User GuideMay 30, 2021
- UI/soundMay 30, 2021
- Game testingMay 18, 2021
- Presentation and GraphicsMay 09, 2021
- Interactions / PuzzlesMay 02, 2021
- Basic Level BlockingApr 25, 2021
- Game Concept LogApr 16, 2021
Leave a comment
Log in with itch.io to leave a comment.